Another way to describe the pathlib
module in Python would be to say that it provides an object-oriented interface for handling filesystem paths. This module simplifies path manipulations, making your code more readable and concise compared to using traditional modules like os
and os.path
.
The name Pathlib
is derived from the term "path library," which is exactly what this module is all about. It provides a set of classes and methods for working with filesystem paths in a more intuitive and object-oriented way.
Overview
The pathlib
module in Python provides an object-oriented interface for handling filesystem paths. It simplifies path manipulations, making your code more readable and concise compared to using traditional modules like os
and os.path
.
Official Documentation
You can find the official documentation for pathlib
at:
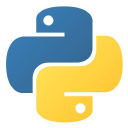
Installation
The pathlib
module is part of Python's standard library and does not require additional installation if you are using Python 3.4 or later. For older versions of Python, you can install it using:
pip install pathlib
Features
- Simplifies working with paths (e.g., file names, directories, extensions).
- Supports handling paths across different operating systems (Windows, macOS, Linux).
- Includes methods for creating, removing, or modifying files and directories.
- Provides an intuitive interface for path iteration and resolution.
Pathlib.Path Methods and Properties
1. Path Components
.name
: Returns the full file name, including the extension.
python Path("/path/to/file.txt").name # "file.txt"
.stem
: Returns the file name without the extension.
python Path("/path/to/file.txt").stem # "file"
.suffix
: Returns the file extension (including the dot).
python Path("/path/to/file.txt").suffix # ".txt"
.suffixes
: Returns a list of all suffixes (useful for files like.tar.gz
).
python Path("/path/to/archive.tar.gz").suffixes # [".tar", ".gz"]
.parent
: Returns the parent directory of the path.
python Path("/path/to/file.txt").parent # "/path/to"
.parts
: Returns all parts of the path as a tuple.
python Path("/path/to/file.txt").parts # ("/", "path", "to", "file.txt")
.anchor
: Returns the root of the path (e.g.,/
on Linux/Mac,C:\
on Windows).
python Path("/path/to/file.txt").anchor # "/"
2. Path Information
.exists()
: Checks if the path exists.
python Path("/path/to/file.txt").exists() # True or False
.is_file()
: Checks if the path is a file.
python Path("/path/to/file.txt").is_file() # True or False
.is_dir()
: Checks if the path is a directory.
python Path("/path/to").is_dir() # True or False
.is_symlink()
: Checks if the path is a symbolic link.
python Path("/path/to/symlink").is_symlink() # True or False
3. Path Manipulation
.with_name(name)
: Returns a newPath
object with the file name replaced.
python Path("/path/to/file.txt").with_name("newfile.txt") # "/path/to/newfile.txt"
.with_suffix(suffix)
: Returns a newPath
object with the file extension replaced.
python Path("/path/to/file.txt").with_suffix(".md") # "/path/to/file.md"
.joinpath(*other)
: Joins the path with one or more subpaths.
python Path("/path/to").joinpath("file.txt") # "/path/to/file.txt"
4. File System Operations
.mkdir(parents=False, exist_ok=False)
: Creates a directory at the path.
python Path("/path/to/newdir").mkdir(parents=True, exist_ok=True)
.rmdir()
: Removes an empty directory.
python Path("/path/to/emptydir").rmdir()
.unlink(missing_ok=False)
: Deletes a file or symbolic link.
python Path("/path/to/file.txt").unlink()
.rename(target)
: Renames or moves the file or directory.
python Path("/path/to/file.txt").rename("/newpath/to/file.txt")
.touch(mode=0o666, exist_ok=True)
: Creates an empty file if it does not exist.
python Path("/path/to/newfile.txt").touch()
5. Iterating Over Directories
.iterdir()
: Iterates over the contents of a directory.
python for item in Path("/path/to").iterdir(): print(item)
.glob(pattern)
: Returns an iterator for paths matching a pattern.
python for file in Path("/path/to").glob("*.txt"): print(file)
.rglob(pattern)
: Returns an iterator for paths matching a pattern recursively.
python for file in Path("/path/to").rglob("*.txt"): print(file)
6. Path Resolution
.absolute()
: Returns the absolute path.
python Path("file.txt").absolute() # "/current/working/dir/file.txt"
.resolve(strict=False)
: Returns the resolved absolute path, resolving symlinks and removing redundant.
and..
.
python Path("file.txt").resolve()
7. Path Conversion
.as_posix()
: Converts the path to a POSIX-style string (slashes).
python Path("C:\\path\\to\\file.txt").as_posix() # "C:/path/to/file.txt"
.as_uri()
: Converts the path to a file URI.
python Path("/path/to/file.txt").as_uri() # "file:///path/to/file.txt"
8. Reading and Writing Files
.read_text(encoding=None)
: Reads the file content as text.
python content = Path("/path/to/file.txt").read_text()
.write_text(data, encoding=None)
: Writes text to the file.
python Path("/path/to/file.txt").write_text("Hello, world!")
.read_bytes()
: Reads the file content as bytes.
python content = Path("/path/to/file.txt").read_bytes()
.write_bytes(data)
: Writes bytes to the file.
python Path("/path/to/file.txt").write_bytes(b"Hello, bytes!")