23 Jul 2022 starting note (Note date indicates latest update.).
Library resources | |
---|---|
PyPI | https://pypi.org/project/requests/ |
Github | https://github.com/psf/requests |
Documentation | https://requests.readthedocs/en/latest/ |
Install with:
pip3 install requests
then:
import requests
POST
url = 'https://www.w3schools.com/python/demopage.php'
myobj = {'somekey': 'somevalue'}
x = requests.post(url, json = myobj)
Sending requests with JSON data and headers
import requests
import json
url = "https://httpbin.org/post"
headers = {"Content-Type": "application/json; charset=utf-8"}
data = {
"id": 1001,
"name": "geek",
"passion": "coding",
}
response = requests.post(url, headers=headers, json=data)
print("Status Code", response.status_code)
print("JSON Response ", response.json())
Example with Grist
response = requests.post(
f"https://docs.getgrist.com/api/docs/{doc_id}/attachments",
files={"upload": open("my_pic.png", "rb")},
headers={"Authorization": f"Bearer {api_key}"},
)
attachment_id = response.json()[0]
Caching sessions
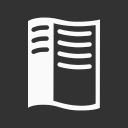
General Usage - requests-cache 0.9.6 documentation
General Usage#
usage
from requests_cache import CachedSession
session = CachedSession()
session.get('http://httpbin.org/get')
to clear cache:
session.cache.clear()
or install globally with:
requests_cache.install_cache()