22 Sep 2022
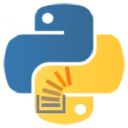
python - Simple way to encode a string according to a password? - Stack Overflow
stackoverflow
my basic script, using an offset in the alphabet:
####################
# Basic String Encryption
test = False
input_raw = 'string to encrypt'
offset = 2 # needs to be <26. 26 returns input_raw
index = {
'a': 1,
'b': 2,
'c': 3,
'd': 4,
'e': 5,
'f': 6,
'g': 7,
'h': 8,
'i': 9,
'j': 10,
'k': 11,
'l': 12,
'm': 13,
'n': 14,
'o': 15,
'p': 16,
'q': 17,
'r': 18,
's': 19,
't': 20,
'u': 21,
'v': 22,
'w': 23,
'x': 24,
'y': 25,
'z': 26,
}
def find_key(input_dict, value):
for k, v in input_dict.items():
if v == value:
return k
def encrypt_with_offset(input,offset=4, test=False):
global index
input = input.lower().strip()
if test:
print(f"\nencrypt_with_offset {input=}\n")
output = ''
for letter in input:
if letter.isalpha(): # check if character is alphabet, else return raw character
letter_index = index[letter]
if test:
print(f"{letter_index=}")
if (letter_index + offset) > len(index):
letter_index = letter_index - 26
encrypted_letter = find_key(index, letter_index + offset)
if test:
print(letter, encrypted_letter)
output = output + encrypted_letter
else:
output = output + letter
if test:
print(f"\n{output=}\n-----------\n")
return output
def decrypt_with_offset(input,offset=4, test=False):
global index
input = input.lower().strip()
if test:
print(f"\ndecrypt_with_offset {input=}\n")
output = ''
for letter in input:
if letter.isalpha(): # check if character is alphabet, else return raw character
letter_index = index[letter]
if test:
print(f"{letter_index=}")
if (letter_index - offset) < 0:
letter_index = letter_index + 26
encrypted_letter = find_key(index, letter_index - offset)
if test:
print(letter, encrypted_letter)
output = output + encrypted_letter
else:
output = output + letter
if test:
print(f"\n{output=}\n-----------\n")
return output
encrypt_output = encrypt_with_offset(input_raw, offset)
print(f"Encrypt: {repr(input_raw)}\toutput with offset {offset}: {repr(encrypt_output)}")
print()
print(f"Decrypt with offset {offset}: {repr(encrypt_output)}\toutput: {repr(decrypt_with_offset(encrypt_output, offset))}")
outputs:
Encrypt: 'string to encrypt' output with offset 2: 'uvtkpi vq gpetarv'
Decrypt with offset 2: 'uvtkpi vq gpetarv' output: 'string to encrypt'
Good enough for my current needs.
improvements:
- add number and special characters to index and logic to scramble further.