30 Jul 2023
Fetching Company data from a Linkedin URL.
Specifically a numerical Linkedin ID.
For example, Salesforce's Linkedin URL with Linkedin ID is https://www.linkedin.com/company/3185
.
I need to get the domain name back from the Linkedin ID.
Using Proxycurl API:
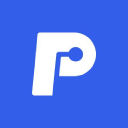
Python code
Using the Company Profile endpoint:
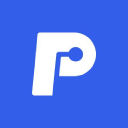
import requests
linkedin_url = 'https://www.linkedin.com/company/3185'
api_endpoint = 'https://nubela.co/proxycurl/api/linkedin/company'
api_key = PROXYCURL_API_TOKEN
header_dic = {'Authorization': 'Bearer ' + api_key}
params = {
'url': linkedin_url,
'resolve_numeric_id': 'true',
# 'categories': 'include',
# 'funding_data': 'include',
# 'extra': 'include',
# 'exit_data': 'include',
# 'acquisitions': 'include',
# 'use_cache': 'if-present',
}
response = requests.get(api_endpoint,
params=params,
headers=header_dic)
print(f"\n{response.status_code=}")
print(f"\n\n{pp.pprint(response.json())}")
print(f"\n\n{response.json()['website']}")
JSON output from API
{ 'acquisitions': None,
'affiliated_companies': [ { 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/company/salesforce-trailhead',
'location': 'San Francisco, California',
'name': 'Trailhead'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-org/',
'location': 'San Francisco, California',
'name': 'Salesforce.org'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-marketing-cloud-/',
'location': None,
'name': 'Salesforce Marketing Cloud'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-admins/',
'location': None,
'name': 'Salesforce Admins'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforcedevs/',
'location': 'San Francisco, California',
'name': 'Salesforce Developers'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-news/',
'location': 'San Francisco, CA',
'name': 'Salesforce News'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://www.linkedin.com/showcase/salesforceevents/',
'location': 'San Francisco, CA',
'name': 'Dreamforce'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/showcase/salesforce-partners/',
'location': 'SAN FRANCISCO, CA',
'name': 'Salesforce Partners'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforcesalescloud/',
'location': None,
'name': 'Salesforce Sales Cloud'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/vlocity-inc',
'location': 'San Francisco, California',
'name': 'Vlocity, a Salesforce Company'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/commercecloud-demandware',
'location': 'San Francisco, California',
'name': 'Salesforce Commerce Cloud'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/pardot',
'location': 'Atlanta, GA',
'name': 'Pardot'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforcecustomersuccessgroup/',
'location': 'San Francisco, California',
'name': 'Salesforce Customer Success'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/showcase/salesforce-architects/',
'location': 'San Francisco, CA',
'name': 'Salesforce Architects'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/showcase/appexchange/',
'location': 'San Francisco, CA',
'name': 'Salesforce AppExchange'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-for-service/',
'location': 'San Francisco, CA',
'name': 'Salesforce for Service'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/exacttarget',
'location': 'Indianapolis, IN',
'name': 'ExactTarget'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-platform/',
'location': 'San Francisco, CA',
'name': 'Salesforce Platform'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/futureforce-salesforce-university-recruiting/',
'location': 'San Francisco, California',
'name': 'Futureforce: Salesforce '
'University Recruiting'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/salesforce-quote-to-cash',
'location': 'San Francisco, CA',
'name': 'Salesforce Revenue Cloud'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-for-small-business/',
'location': 'San Francisco, CA',
'name': 'Salesforce for Small Business'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-for-government/',
'location': 'Reston, Virginia',
'name': 'Salesforce for Government'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-industries/',
'location': 'San Francisco, CA',
'name': 'Salesforce Industries'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/salesforce-quip',
'location': None,
'name': 'Salesforce Quip'},
{ 'industry': 'Information Services',
'link': 'https://www.linkedin.com/company/salesforce-trailhead-superbadges',
'location': None,
'name': 'Salesforce Trailhead Superbadges'},
{ 'industry': 'Professional Training and '
'Coaching',
'link': 'https://www.linkedin.com/school/salesforcemilitary/',
'location': 'San Francisco, California',
'name': 'Salesforce Military'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/school/salesforce-pathfinder-training-program/',
'location': 'San Francisco, CA',
'name': 'Salesforce Pathfinder Training '
'Program'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://ca.linkedin.com/company/workdotcom',
'location': 'Toronto, Ontario',
'name': 'Salesforce Work.com'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/company/model-metrics',
'location': 'Chicago, IL',
'name': 'Model Metrics'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/clipboard-inc-',
'location': 'Bellevue, WA',
'name': 'Clipboard, inc.'},
{ 'industry': None,
'link': 'https://www.linkedin.com/showcase/tableau-crm/',
'location': None,
'name': 'Tableau CRM'},
{ 'industry': None,
'link': 'https://www.linkedin.com/showcase/data-com/',
'location': None,
'name': 'Data.com'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/showcase/salesforce-experience-cloud/',
'location': 'San Francisco, California',
'name': 'Salesforce Experience Cloud'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://www.linkedin.com/company/manymoon',
'location': None,
'name': 'Manymoon'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://ca.linkedin.com/company/goinstant',
'location': None,
'name': 'GoInstant'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/showcase/salesforce.org-partners/',
'location': None,
'name': 'SalesforceOrg Partners'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/company/edgespring',
'location': 'San Mateo, CA',
'name': 'EdgeSpring (acquired by '
'Salesforce)'},
{ 'industry': 'Software Development',
'link': 'https://fr.linkedin.com/company/entropysoft',
'location': 'Paris, IDF',
'name': 'EntropySoft'},
{ 'industry': 'Software Development',
'link': 'https://ca.linkedin.com/company/sitemasher',
'location': 'Vancouver, British Columbia',
'name': 'Sitemasher'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://il.linkedin.com/company/navajo-systems',
'location': None,
'name': 'Navajo Systems'},
{ 'industry': 'Computer Networking Products',
'link': 'https://www.linkedin.com/company/etacts-inc.',
'location': None,
'name': 'Etacts Inc.'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/company/bluetail',
'location': None,
'name': 'BlueTail'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/thinkfuse',
'location': 'Seattle, WA',
'name': 'Thinkfuse'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://www.linkedin.com/company/plannedup',
'location': 'Boston, MA',
'name': 'Ourglass.'},
{ 'industry': 'Software Development',
'link': 'https://ca.linkedin.com/company/radian6-technologies',
'location': None,
'name': 'Radian6 Technologies'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/groupswim',
'location': 'San Francisco, CA',
'name': 'GroupSwim'},
{ 'industry': 'Wireless Services',
'link': 'https://www.linkedin.com/company/sendia-corporation',
'location': None,
'name': 'Sendia Corporation'},
{ 'industry': 'Software Development',
'link': 'https://fr.linkedin.com/company/instranet',
'location': None,
'name': 'InStranet'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://www.linkedin.com/company/stypi',
'location': None,
'name': 'Stypi'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/prior-knowledge-inc-',
'location': None,
'name': 'Prior Knowledge, Inc.'},
{ 'industry': 'Technology, Information and '
'Internet',
'link': 'https://www.linkedin.com/company/activa-live',
'location': None,
'name': 'Activa Live'}],
'background_cover_image_url': 'https://s3.us-west-000.backblazeb2.com/proxycurl/company/salesforce/cover?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=0004d7f56a0400b0000000001%2F20230730%2Fus-west-000%2Fs3%2Faws4_request&X-Amz-Date=20230730T200318Z&X-Amz-Expires=3600&X-Amz-SignedHeaders=host&X-Amz-Signature=16fbd3214b47fe9da583b83a3bf14169551a2c9419400f0b52bb424767bade34',
'categories': None,
'company_size': [10001, None],
'company_size_on_linkedin': 71750,
'company_type': 'PUBLIC_COMPANY',
'description': '๐ Weโre Salesforce, the Customer Company.\n'
'AI + Data + CRM = Customer Magic. โจ \n'
'\n'
'Privacy Statement: '
'http://www.salesforce.com/company/privacy/',
'exit_data': None,
'extra': None,
'follower_count': 4650650,
'founded_year': None,
'funding_data': None,
'hq': { 'city': 'San Francisco',
'country': 'US',
'is_hq': True,
'line_1': '1 Market',
'postal_code': '94105',
'state': 'CA'},
'industry': 'Software Development',
'linkedin_internal_id': '3185',
'locations': [ { 'city': 'San Francisco',
'country': 'US',
'is_hq': True,
'line_1': '1 Market',
'postal_code': '94105',
'state': 'CA'},
{ 'city': 'Staines',
'country': 'CH',
'is_hq': False,
'line_1': '1 Market',
'postal_code': None,
'state': 'Switzerland'},
{ 'city': 'Singapore',
'country': 'US',
'is_hq': False,
'line_1': '1 Market',
'postal_code': '03898',
'state': 'Singapore 9'},
{ 'city': 'Tokyo',
'country': 'US',
'is_hq': False,
'line_1': '1 Market',
'postal_code': None,
'state': 'Japan 106-6139'},
{ 'city': 'Munich',
'country': 'DE',
'is_hq': False,
'line_1': 'Erika-Mann.Straรe 63',
'postal_code': None,
'state': None},
{ 'city': 'London',
'country': 'GB',
'is_hq': False,
'line_1': '9 Bishopsgate',
'postal_code': None,
'state': 'England EC2N 3'},
{ 'city': 'Melbourne',
'country': 'AU',
'is_hq': False,
'line_1': '55 Collins St',
'postal_code': '3000',
'state': 'VIC'},
{ 'city': 'Sydney',
'country': 'AU',
'is_hq': False,
'line_1': '201 Sussex St',
'postal_code': '2000',
'state': 'NSW'},
{ 'city': 'Paris',
'country': 'FR',
'is_hq': False,
'line_1': '3 Avenue Octave Greard',
'postal_code': '75007',
'state': 'IdF'},
{ 'city': 'Chicago',
'country': 'US',
'is_hq': False,
'line_1': '111 W Illinois St',
'postal_code': '60654',
'state': 'IL'},
{ 'city': 'Dallas',
'country': 'US',
'is_hq': False,
'line_1': '14902 Preston Rd',
'postal_code': '75254',
'state': 'TX'},
{ 'city': 'Atlanta',
'country': 'US',
'is_hq': False,
'line_1': '950 E Paces Ferry Rd NE',
'postal_code': '30326',
'state': 'GA'},
{ 'city': 'Burlington',
'country': 'US',
'is_hq': False,
'line_1': '5 Wall St',
'postal_code': '01803',
'state': 'MA'},
{ 'city': 'Milan',
'country': 'IT',
'is_hq': False,
'line_1': 'Via Niccolo Copernico, 38',
'postal_code': '20125',
'state': 'Lomb.'},
{ 'city': 'Berlin',
'country': 'DE',
'is_hq': False,
'line_1': 'Kurfurstendamm 194',
'postal_code': '10707',
'state': 'BE'},
{ 'city': 'Madrid',
'country': 'ES',
'is_hq': False,
'line_1': 'Paseo de la Castellana, 79',
'postal_code': '28046',
'state': 'Community of Madrid'},
{ 'city': 'Tel Aviv-Yafo',
'country': 'IL',
'is_hq': False,
'line_1': 'Derekh Menahem Begin 154',
'postal_code': '60000',
'state': 'Tel Aviv'}],
'name': 'Salesforce',
'profile_pic_url': 'https://s3.us-west-000.backblazeb2.com/proxycurl/company/salesforce/profile?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=0004d7f56a0400b0000000001%2F20230730%2Fus-west-000%2Fs3%2Faws4_request&X-Amz-Date=20230730T200318Z&X-Amz-Expires=3600&X-Amz-SignedHeaders=host&X-Amz-Signature=cda609cea12c16987c544972bf09a95e7e3b31da6e1748a2f6140bf9e2049a22',
'search_id': '3185',
'similar_companies': [ { 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/google',
'location': 'Mountain View, CA',
'name': 'Google'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/microsoft',
'location': 'Redmond, Washington',
'name': 'Microsoft'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/tiny-spec-inc',
'location': 'San Francisco, California',
'name': 'Slack'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/amazon',
'location': 'Seattle, WA',
'name': 'Amazon'},
{ 'industry': 'Software Development',
'link': 'https://au.linkedin.com/company/atlassian',
'location': 'Sydney, NSW',
'name': 'Atlassian'},
{ 'industry': 'IT Services and IT Consulting',
'link': 'https://www.linkedin.com/company/oracle',
'location': 'Austin, Texas',
'name': 'Oracle'},
{ 'industry': 'Business Consulting and Services',
'link': 'https://www.linkedin.com/company/deloitte',
'location': None,
'name': 'Deloitte'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/meta',
'location': 'Menlo Park, CA',
'name': 'Meta'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/adobe',
'location': 'San Jose, CA',
'name': 'Adobe'},
{ 'industry': 'Software Development',
'link': 'https://www.linkedin.com/company/linkedin',
'location': 'Sunnyvale, CA',
'name': 'LinkedIn'}],
'specialities': [ 'Enterprise Cloud Computing and Customer Relationship '
'Management'],
'tagline': '๐ Weโre Salesforce, the Customer Company.\n'
'AI + Data + CRM = Customer Magic. โจ',
'universal_name_id': 'salesforce',
'updates': [ { 'article_link': None,
'image': None,
'posted_on': {'day': 13, 'month': 7, 'year': 2023},
'text': 'Companies are scrambling to hire people that '
'have the skills to put generative AI to work. '
'Hereโs how you can quickly skill up and get '
'AI-ready. \n'
'\n'
'๐๏ธ Subscribe for more tips to help you succeed '
'in an AI-first world. #AskMoreOfAI',
'total_likes': 1172},
{ 'article_link': None,
'image': None,
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': 'Worried about whether generative AI is here to '
'replace your sales reps? Fear not. Weโre '
'diving into all the advantages of AI and how '
'it can benefit your sales teams. RSVP to join '
'the conversation on July 26, right here on '
'LinkedIn.',
'total_likes': 13},
{ 'article_link': 'https://www.salesforce.com/blog/smb-automation-benefits-of-generative-ai/?',
'image': None,
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': 'Sales reps, meet your new AI-powered ally! '
'From prospecting emails to personalizing '
'engagements, explore how generative AI will '
'help your teams sell smarter: '
'https://sforce.co/3rywAzF',
'total_likes': 44},
{ 'article_link': 'https://www.salesforce.com/news/stories/sales-gpt-service-gpt-ga/',
'image': None,
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': None,
'total_likes': 0},
{ 'article_link': 'https://www.salesforce.com/news/stories/sales-gpt-service-gpt-ga/',
'image': None,
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': None,
'total_likes': 0},
{ 'article_link': None,
'image': 'https://media.licdn.com/dms/image/D5610AQHVCktqWoIwTQ/image-shrink_1280/0/1689796814021?e=1690444800&v=beta&t=7QkEdZA6SQSrm7HPqLvjxoRG2r383UjBdu5fDUUQvsY',
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': '๐ Your AI learning journey starts here. Get '
'started with #EinsteinGPT โ free on Trailhead: '
'https://sforce.co/3LiY3Np',
'total_likes': 129},
{ 'article_link': None,
'image': None,
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': 'AI + your service agents = dream team. ๐ค Give '
'your contact center an upgrade with generative '
'AI: https://sforce.co/3PXfuWe',
'total_likes': 115},
{ 'article_link': None,
'image': 'https://media.licdn.com/dms/image/D5610AQEV7xi48GTWFw/videocover-high/0/1689790077054?e=1690444800&v=beta&t=QN40L7r1LjkTAA3GttJWBSySQOayuF3-g3lE_uc_658',
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': '#SalesGPT and #ServiceGPT are HERE. And with '
'every AI feature built on our #EinsteinGPT '
'Trust Layer โ all data is handled with trust. '
'๐ฆพ๐\n'
'\n'
'See it in action. ๐ https://sforce.co/3XWRyEc',
'total_likes': 1656},
{ 'article_link': None,
'image': 'https://media.licdn.com/dms/image/D5610AQFqThF-elttFg/image-shrink_800/0/1689789621988?e=1690444800&v=beta&t=6gIZFkYtkx4t3604eZ98wgGwokffPoR-CI4w-eiR4WY',
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': '#ServiceGPT is now GA โ and everything you '
'need to know is on Trailhead: '
'https://sforce.co/3Y1joiY',
'total_likes': 43},
{ 'article_link': None,
'image': 'https://media.licdn.com/dms/image/D5610AQGcAMxRj_XvTQ/image-shrink_800/0/1689786481825?e=1690444800&v=beta&t=90tx8R49xx0BT-gnx_WvK1QFFrlaQbBVtttkzAaV4C4',
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': 'Hey, #Salesblazer! Your super sales sidekick '
'is here: #SalesGPT is now GA. โก๏ธ\n'
'\n'
'Start learning on Trailhead: '
'https://sforce.co/3Q2k1qg',
'total_likes': 146},
{ 'article_link': None,
'image': 'https://media.licdn.com/dms/image/D5610AQG1SOhRIchS6Q/image-shrink_1280/0/1689782426614?e=1690444800&v=beta&t=T6nNnflGvls_UM4z_DsuGgzBE67oAzOhRI6MKVk4T1w',
'posted_on': {'day': 19, 'month': 7, 'year': 2023},
'text': 'Can a career in the tech industry make a '
'positive impact on the planet? Yes ๐ฑ Learn '
'firsthand how our customer success teams help '
'reduce our customersโ carbon footprint as '
'leaders share five takeaways on how you can '
'align your career with your passion.\n'
'\n'
'https://sforce.co/3r7J94D',
'total_likes': 51}],
'website': 'http://www.salesforce.com'}