Viewport
The viewport defines the visible area of the device the site is accessed from.
Add a viewport in <head>
as:
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0" />
Layout
3 ways:
- Float layouts (old, dismiss)
- Flexbox (1-dimensional rows, for simple components layout)
- CSS grid (2-dimensional grid, for page layouts and complex components)
Flexbox
CSS grid
grid containers
display: grid;
/* number and size of columns and rows */
grid-template-columns: 3fr auto 1fr 2fr; /* 2 fixed columns, 2 responsive columns. `fr` for fractional */
grid-template-rows: 300px 200px ; /* sets rows heights */
/* gaps / gutters */
column-gap: 30px;
row-gap: 50px;
/* alignment */
justify-items: stretch | start | center | end;
align-items: stretch | start | center | end;
repeat function
grid-template-columns: repeat(16, 1fr); /* for 16 columns, each 1fr */
align within containers
/* cell alignment */
justify-content: center | space-between;
align-content: center;
/* items alignment within cells */
align-items: center | stretch | start | end; /* stretch is default */
justify-items: center; /* stretch is default */
grid items
/* to place a grid item into a specific cell or group of cells */
.el-1 {
grid-column: <start line> / <end line> | span <number>;
grid-row: <start line> / <end line> | span <number>;
}
eg
.el-1 {
grid column: 1 / -1; /* -1 to span the rest of the space, eg full row here */
grid row: 2 / 3;
}
align within grid items
.el-1 {
align-self: center;
justify-self: center;
}
CSS
media queries
Use @media
to change properties based on viewport size.
Mobile first means changing properties if viewport is larger than mobile:
/* For mobile phones: */
[class*="col-"] {
width: 100%;
}
@media only screen and (min-width: 600px) {
/* For tablets: */
.col-s-1 {width: 8.33%;}
.col-s-2 {width: 16.66%;}
.col-s-3 {width: 25%;}
.col-s-4 {width: 33.33%;}
.col-s-5 {width: 41.66%;}
.col-s-6 {width: 50%;}
.col-s-7 {width: 58.33%;}
.col-s-8 {width: 66.66%;}
.col-s-9 {width: 75%;}
.col-s-10 {width: 83.33%;}
.col-s-11 {width: 91.66%;}
.col-s-12 {width: 100%;}
}
@media only screen and (min-width: 768px) {
/* For desktop: */
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
ideally:
/* Extra small devices (phones, 600px and down) */
@media only screen and (max-width: 600px) {...}
/* Small devices (portrait tablets and large phones, 600px and up) */
@media only screen and (min-width: 600px) {...}
/* Medium devices (landscape tablets, 768px and up) */
@media only screen and (min-width: 768px) {...}
/* Large devices (laptops/desktops, 992px and up) */
@media only screen and (min-width: 992px) {...}
/* Extra large devices (large laptops and desktops, 1200px and up) */
@media only screen and (min-width: 1200px) {...}
to handle orientation:
@media only screen and (orientation: landscape) {
body {
background-color: lightblue;
}
}
hide elements
/* If the screen size is 600px wide or less, hide the element */
@media only screen and (max-width: 600px) {
div.example {
display: none;
}
}
variable font-size
/* If the screen size is 601px or more, set the font-size of <div> to 80px */
@media only screen and (min-width: 601px) {
div.example {
font-size: 80px;
}
}
/* If the screen size is 600px or less, set the font-size of <div> to 30px */
@media only screen and (max-width: 600px) {
div.example {
font-size: 30px;
}
}
Typefaces
16px to 32px for most text. Titles can be bigger, eg 85px.
Font weight 400 or more.
<75 characters per line.
line height 1.5 or 2 (1.5 for big text)
All caps for short titles
Images
img {
width: 100%;
height: auto;
}
Colors
Main, Accent and Grey.
Tints are lighter, shades are darker.
Use:
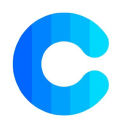
or
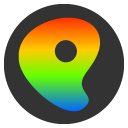
Frameworks
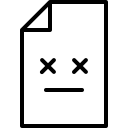
- good candidate, like the style, but perhaps too bare bones?
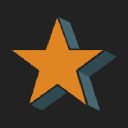
- this is the one I started with. Lacks grid system and more though. Too bare bones for my needs.
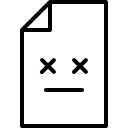
- looks comprehensive, but not a fan of the elements' design.
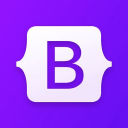
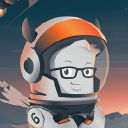
See all elements: https://get/sites/docs/kitchen-sink.html#accordion
Design resources
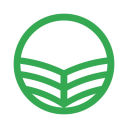
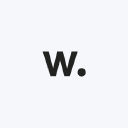
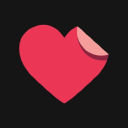
Demos
approach to implement
with collapsing rows while maintaining headers:
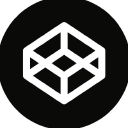
Resources
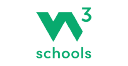
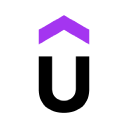